Easy way to learn Swift (IOS)
Swift (IOS)
Course Description
Swift is a new programming language developed by Apple Inc for iOS and (Mac) OS X development. Swift adopts the best of C and Objective-C, without the constraints of C compatibility.
- Swift makes use of safe programming patterns.
- Swift provides modern programming features.
- Swift provides Objective-C like syntax.
- Swift is a fantastic way to write iOS and OS X apps.
- Swift provides seamless access to existing Cocoa
frameworks. - Swift unifies the procedural and object-oriented portions
of the language.Swift does not need a separate library import to support
functionalities like input/output or string handling.Swift uses the same runtime as the existing Obj-C system on Mac OS and iOS, which enables Swift programs to run on many existing iOS 6 and OS X 10.8 platforms. Swift comes with playground feature where Swift programmers can write their code and execute it to see the results
immediately.The first public release of Swift was released in 2010. It took Chris Lattner almost 14 years to come up with the first official version, and later, many other contributors supported
it. Swift has been included in Xcode 6 beta.Swift designers took ideas from various other popular languages such as Objective-C, Rust, Haskell, Ruby, Python, C#, and CLU.
More About Swift
Apple laid the foundation for Swift by advancing our existing compiler, debugger, and framework infrastructure. Swift developer simplified the memory management with Automatic Reference Counting (ARC) which you will study in details later. The framework on which Swift is based is built on a solid base of foundation and has been modernized and standardized throughout. Swift is familiar to Objective-C developers and adopts the readability of Objective-C's named parameter and the power of DOM (Dynamic Object Model) of Objective-C. Building from this common ground, Swift brings in many new features and unifies the procedural and object-oriented concepts of the language. This new programming language was released in the year 2010. Chris Lattner took almost fourteen years to come up with the first official version of Swift. Later, it was supported by many other contributions. Swift has been included in Xcode 6 beta.
Basic Syntax
Hello World in Swift
Example :-
This is the first simple program using Swift:
This is the first simple program using Swift:
import UIKit
var str = "Hello World!"
print(str)
OUTPUT
Hello World!
Tokens in Swift
A Swift program consists of various tokens and a token is a keyword, an identifier, a constant, a string literal, or a symbol. For example,
the following Swift statement consists of three tokens:
import UIKit
print("test!")
/* The individual tokens are :
print(
"test!"
)
Comments
Comments are those lines in Swift program which do not get compile by the Swift compiler at the time of compilation. They act as helping texts for programmers to understand the working of the code snippet and are ignored by the compiler. Multiline comments start with /* and terminate with */ and in between the strings that will act as comment.
import UIKit
/* This is the comment section */
var str = "Hello Friends"
print(str)
/* This is another comment :: end of code */
Multiline comments can be nested like this:
/* My first program in Swift!
/* Hello, Shivan */
Single line comments can be written using double slash.
Example:
// Hello Shivan.
Semicolons
Swift does not require you to type a semicolon (;) after each statement in your code, though it’s optional; and if you use a semicolon, then the compiler does not complain about it. However, if you are using multiple statements in the same line, then it is required to use a semicolon as a delimiter, otherwise the compiler will raise a syntax error. You can
write the above Hello, World! Program as follows:
write the above Hello, World! Program as follows:
import UIKit
var myString = "Hello, Good Mornimg!";
print(myString)
Identifiers
A Swift identifier is a name used to identify a variable, function, or any other user defined item. An identifier starts
with an alphabet A to Z or a to z or an underscore _ followed by zero or more letters, underscores, and digits (0 to 9).
Swift does not allow special characters such as @, $, and % within identifiers. Swift is a case sensitive programming language. Thus, Manpower and manpower are two different identifiers in Swift.
with an alphabet A to Z or a to z or an underscore _ followed by zero or more letters, underscores, and digits (0 to 9).
Swift does not allow special characters such as @, $, and % within identifiers. Swift is a case sensitive programming language. Thus, Manpower and manpower are two different identifiers in Swift.
Here are some examples of acceptable identifiers:
myname50 _temp j a23b9 retVal
To use a reserved word as an identifier, you will need to put a backtick (`) before and after it. For example, class is not
a valid identifier, but `class` is valid.
a valid identifier, but `class` is valid.
Whitespaces
A line containing only whitespace, possibly with a comment, is known as a blank line, and a Swift compiler totally ignores it.
Whitespace is the term used in Swift to describe blanks, tabs, newline characters, and comments. Whitespaces separate one part of a statement from another and enable the compiler to identify where one element in a statement, such as int, ends and the next element begins.
Whitespace is the term used in Swift to describe blanks, tabs, newline characters, and comments. Whitespaces separate one part of a statement from another and enable the compiler to identify where one element in a statement, such as int, ends and the next element begins.
Therefore, in the following statement:
var age
There must be at least one whitespace character (usually a space) between var and age for the compiler to be able to distinguish them. On the other hand, in the following statement:
int fruit = apples + oranges //get the total fruits
No whitespace characters are necessary between fruit and =, or between = and apples, although you are free to include some for better readability.
Literals
A literal is the source code representation of a value of an integer, floating-point number, or string type. The following
are examples of literals:
are examples of literals:
92 // Integer literal
4.24159 // Floating-point literal
"Hello, World!" // String literal
Data Types
Built-in Data Types
Swift offers the programmer a rich assortment of built-in as well as user-defined data types. The following types of basic data types are most frequently when declaring variables −
- Int or UInt − This is used for whole numbers. More specifically, you can use Int32, Int64 to define 32 or 64 bit signed integer, whereas UInt32 or UInt64 to define 32 or 64 bit unsigned integer variables. For example, 42 and -23.
- Float − This is used to represent a 32-bit floating-point number and numbers with smaller decimal points. For example, 3.14159, 0.1, and -273.158.
- Double − This is used to represent a 64-bit floating-point number and used when floating-point values must be very large. For example, 3.14159, 0.1, and -273.158.
- Bool − This represents a Boolean value which is either true or false.
- String − This is an ordered collection of characters. For example, “Hello, World!”
- Character − This is a single-character string literal. For example, “C”
- Optional − This represents a variable that can hold either a value or no value.
We have listed here a few important points related to Integer types −
- On a 32-bit platform, Int is the same size as Int32.
- On a 64-bit platform, Int is the same size as Int64.
- On a 32-bit platform, UInt is the same size as UInt32.
- On a 64-bit platform, UInt is the same size as UInt64.
- Int8, Int16, Int32, Int64 can be used to represent 8 Bit, 16 Bit, 32 Bit, and 64 Bit forms of signed integer.
- UInt8, UInt16, UInt32, and UInt64 can be used to represent 8 Bit, 16 Bit, 32 Bit and 64 Bit forms of unsigned integer.
Bound Values
The following table shows the variable type, how much memory it takes to store the value in memory, and what is the maximum and minimum value which can be stored in such type of variables.
Type Aliases
Type | Typical Bit Width | Typical Range |
---|---|---|
Int8 | 1byte | -127 to 127 |
UInt8 | 1byte | 0 to 255 |
Int32 | 4bytes | -2147483648 to 2147483647 |
UInt32 | 4bytes | 0 to 4294967295 |
Int64 | 8bytes | -9223372036854775808 to 9223372036854775807 |
UInt64 | 8bytes | 0 to 18446744073709551615 |
Float | 4bytes | 1.2E-38 to 3.4E+38 (~6 digits) |
Double | 8bytes | 2.3E-308 to 1.7E+308 (~15 digits) |
You can create a new name for an existing type using typealias. Here is the simple syntax to define a new type using typealias −
typealias newname = type
For example, the following line instructs the compiler that Feet is another name for Int −
typealias Feet = Int
Now, the following declaration is perfectly legal and creates an integer variable called distance −
import UIKit
typealias Feet = Int
var distance: Feet = 200
print(distance)
When we run the above program using playground, we get the following result −
OUTPUT :-
200
Type Safety
Swift is a type-safe language which means if a part of your code expects a String, you can’t pass it an Int by mistake.
As Swift is type-safe, it performs type-checks when compiling your code and flags any mismatched types as errors.
import UIKit
var A = 42
A = "This is hello"
print(A)
When we compile the above program, it produces the following compile time error.
OUTPUT:-
Playground execution failed: error: <EXPR>:6:6: error: cannot assign to 'let' value 'A'
A = "This is hello"
Type Inference
Type inference enables a compiler to deduce the type of a particular expression automatically when it compiles your code, simply by examining the values you provide. Swift uses type inference to work out the appropriate type as follows.
import UIKit
// varA is inferred to be of type Int
var varA = 42
print(varA)
// varB is inferred to be of type Double
var varB = 3.14159
print(varB)
// varC is also inferred to be of type Double
var varC = 3 + 0.14159
print(varC)
When we run the above program using playground, we get the following result −
OUTPUT:-
42
3.14159
3.14159
Variables
Swift supports the following basic types of variables:
- Int or UInt – This is used for whole numbers. More specifically, you can use Int32, Int64 to define 32 or 64
bit signed integer, whereas UInt32 or UInt64 to define 32 or 64 bit unsigned integer variables. For example, 42 and -23. - Float – This is used to represent a 32-bit floating-point number. It is used to hold numbers with smaller decimal
points. For example, 3.14159, 0.1, and -273.158. - Double – This is used to represent a 64-bit floating- point number and used when floating-point values must be
very large. For example 3.14159, 0.1, and -273.158. - Bool – This represents a Boolean value, which is either true or false.
- String – This is an ordered collection of characters. For example, “Hello, World!”
- Character – This is a single-character string literal. For example, “C”
A variable provides us with named storage that our programs can operate. Variable names cannot contain mathematical symbols, arrows, private-use (or invalid) Unicode code points, or line- and box-drawing characters nor can they begin with a number, although numbers may be included elsewhere within the name.
Naming Variables
The name of a variable can be composed of letters, digits, and the underscore character. It must begin with either a letter
or an underscore. Upper and lowercase letters are distinct because Swift is a case-sensitive programming language.
or an underscore. Upper and lowercase letters are distinct because Swift is a case-sensitive programming language.
You can use simple or Unicode characters to name your variables. The following example shows how you can name the
variables:
variables:
var name = "Shivan"
print(name)
OUTPUT:-
Shivan
Variable Declaration
A variable declaration tells the compiler of which type, where and how much to create the storage for the variable. Declarations of variables are done using the varkeyword.
Example:
import UIKit
var firstvar = 68
println(firstvar)
The output of the above code snippet will be:
OUTPUT:-
68
Type Annotations
Programmers can supply a type annotation when they declare a variable, to be clear about the kind of value(s) that the declared variable can store. The general form of using this variable is:
Syntax:
var variableName:<data type> = <optional initial value>
Example:
import UIKit
var firstvar = 68
print(firstvar)
var secVar:Float
secVar = 3.14159
print (secVar)
print(" 1st Value \(firstvar) 2nd Value \(secVar) ")
OUTPUT:-
68
3.14159
1st Value 68 2nd Value 3.14159
Printing Variables
OUTPUT:-
68
3.14159
1st Value 68 2nd Value 3.14159
Printing Variables
You can print the current value of a constant or variable with the println function. You can interpolate a variable value by wrapping the name in parentheses and escape it with a backslash before the opening parenthesis: Following are valid
Example:-
var varA = "Godzilla"
var varB = 1000.00
print("Value of \(varA) is more than \(varB) millions")
OUTPUT:-
Value of Godzilla is more than 1000.0 millions
Optionals
Swift also introduces Optionals type, which handles the absence of a value. Optionals say either “there is a value, and it equals x” or “there isn’t a value at all”.
An Optional is a type on its own, actually one of Swift’s new super-powered enums. It has two possible values, None and Some(T), where Tis an associated value of the correct data type available in Swift.
Here’s an optional Integer declaration −
var perhapsInt: Int?
Here’s an optional String declaration −
var perhapsStr: String?
The above declaration is equivalent to explicitly initializing it to nil which means no value
var perhapsStr: String? = nil
Let’s take the following example to understand how optionals work in Swift −var myString:String? = nil
import UIKit if str != nil { print(str) } else { print("String has nil value") }
When we run the above program using playground, we get the following result −
String has nil value
Optionals are similar to using nil with pointers in Objective-C, but they work for any type, not just classes.
Forced Unwrapping
If you defined a variable as optional, then to get the value from this variable, you will have to unwrap it. This just means putting an exclamation mark at the end of the variable.
Let’s take a simple example −
Live Demo
var str:String? str = "Hello, Shivan!" if str != nil { print(str) } else { print("String has nil value") }
When we run the above program using playground, we get the following result −
Optional("Hello, Shivan!")
Now let’s apply unwrapping to get the correct value of the variable −
var str:String? str = "Hello, Friends!" if str != nil { print(str!) } else { print("myString has nil value") }
When we run the above program using playground, we get the following result −
Hello, Friends!
Automatic Unwrapping
You can declare optional variables using exclamation mark instead of a question mark. Such optional variables will unwrap automatically and you do not need to use any further exclamation mark at the end of the variable to get the assigned value. Let’s take a simple example −var myString:String!
let str = "Hello, Friends!" if str != nil { print(str) } else { print("myString has nil value") }
When we run the above program using playground, we get the following result −
Hello, Friends!
Optional Binding
Use optional binding to find out whether an optional contains a value, and if so, to make that value available as a temporary constant or variable.
An optional binding for the if statement is as follows −
if let constantName = someOptional {
statements
}
Let’s take a simple example to understand the usage of optional binding −
var str:String? str = "Hello, Friends!" if let str2 = str { print("Your new String has - \(str2)") } else { print("Your string does not have a value") }
When we run the above program using playground, we get the following result −
Your new String has - Hello, Friends!
Constants
Constants refer to fixed values that a program may not alter during its execution. Constants can be of any of the basic
data types like an integer constant, a floating constant, a character constant, or a string literal. There are enumeration
constants as well.
data types like an integer constant, a floating constant, a character constant, or a string literal. There are enumeration
constants as well.
Constants are treated just like regular variables except the fact that their values cannot be modified after their
definition.
definition.
Naming Constants
Example:
let _const = "Hello, Swift!"
print(_const)
Constants Declaration
Before you use constants, you must declare them using let keyword as follows:
let constantName = <initial value>
Following is a simple example to show how to declare a constant in Swift:
Example:
let constantName = <initial value>
Here is a sample Swift Program
Example:
You can provide a type annotation when you declare a constant,to be clear about the kind of values the constant can store.Following is the syntax
let constantName:<data type> = <optional initial value>
The following example shows how to declare a constant in Swift using Annotation. Here it is important to note that it is
mandatory to provide an initial value while creating a constant:
mandatory to provide an initial value while creating a constant:
let const:Float = 3.14159print(const)
Printing Constants
You can print the current value of a constant or variable using print function. You can interpolate a variable value
by wrapping the name in parentheses and escape it with a backslash before the opening parenthesis. Following are valid
examples:
by wrapping the name in parentheses and escape it with a backslash before the opening parenthesis. Following are valid
examples:
let constA = "Godzilla"let constB = 1000.00print("Value of \(constA) is more than \(constB) millions")
Literals
In most of the programming languages, literals and constants play a major role in dealing with values within a program. Swift also support the concept of literals and constants. They are used to hold a constant value for expressing them within the code and hold a memory location. In this chapter you will learn about how you can use constants and literals in Swift programming language.
The following are examples of literals −
42 // Integer literal3.14159 // Floating-point literal"Hello, world!" // String literal
What are Literals?
Literals are most obvious kinds of constants and are used to express particular values within the source code of the program. In other words, it is the source code representation of a value. Here are the lists of some of the literals that are valid in Swift -
Swift Literals are of various types. They are:
Numeric Literals
It has its sub categories as integer and floating point literals and can be written as:
All the above literals have a decimal value of 17. It is to be noted that floating point literals can also be decimal with no prefix or hexadecimal literal with a 0x prefix. They must always have a number on both side of the decimal point. They can also contain an optional exponent which is indicated by the upper case or lower case latter e and / or upper case or lower case letter p in case of hexadecimal floats.
For decimal numbers with an exponent of exp, the base number is multiplied by 10exp:
1.25e2 means 1.25 × 102, or 125.0.
1.25e-2 means 1.25 × 10-2, or 0.0125.
For hexadecimal numbers with an exponent of exp, the base number is multiplied by 2exp:
0xFp2 means 15 × 22, or 60.0.
0xFp-2 means 15 × 2-2, or 3.75.
Integer Literals
An integer literal can be a decimal, binary, octal, or hexadecimal constant. Binary literals begin with 0b, octal literals begin with 0o, and hexadecimal literals begin with 0x and nothing for decimal.
Here are some examples of integer literals −
let hexadecimalInteger = 0x11 // 17 in hexadecimal notationlet decimalInteger = 17 // 17 in decimal notationlet binaryInteger = 0b10001 // 17 in binary notationlet octalInteger = 0o21 // 17 in octal notationlet hexadecimalInteger = 0x11 // 17 in hexadecimal notation
Floating-point Literals
A floating-point literal has an integer part, a decimal point, a fractional part, and an exponent part. You can represent floating point literals either in decimal form or hexadecimal form.
Decimal floating-point literals consist of a sequence of decimal digits followed by either a decimal fraction, a decimal exponent, or both.
Hexadecimal floating-point literals consist of a 0x prefix, followed by an optional hexadecimal fraction, followed by a hexadecimal exponent.
Here are some examples of floating-point literals −
let decimalDouble = 12.1875let exponentDouble = 1.21875e1let hexadecimalDouble = 0xC.3p0
String Literals
A string literal is a sequence of characters surrounded by double quotes, with the following form −
"characters"
String literals cannot contain an unescaped double quote (“), an unescaped backslash (\), a carriage return, or a line feed. Special characters can be included in string literals using the following escape sequences −
The following example shows how to use a few string literals −
Escape sequence | Meaning |
---|---|
\0 | Null Character |
\\ | \character |
\b | Backspace |
\f | Form feed |
\n | Newline |
\r | Carriage return |
\t | Horizontal tab |
\v | Vertical tab |
\' | Single Quote |
\" | Double Quote |
\000 | Octal number of one to three digits |
\xhh... | Hexadecimal number of one or more digits |
import UIKitlet string = "Hello\tShivan\n\nHello\'Swift\'"print(string)
When we run the above program using playground, we get the following result −
Hello ShivanHello'Swift'
Boolean Literals
There are three Boolean literals and they are part of standard Swift keywords −
- A value of true representing true.
- A value of false representing false.
- A value of nil representing no value.
Operators
An operator is a symbol that tells the compiler to perform specific mathematical or logical manipulations. Objective-C is rich in built-in operators and provides the following
Types of Swift Operators
Swift is rich in operators and provides the following types of operators:
Arithmetic Operators
This tutorial will explain the arithmetic, relational, logical, bitwise, assignment and other operators one by one.
Arithmetic Operators
The following table shows all the arithmetic operators supported by Swift language. Assume variable A holds 10 and variable B holds 20, then −
Comparison Operators
Operator | Description | Example |
---|---|---|
+ | Adds two operands | A + B will give 30 |
− | Subtracts second operand from the first | A − B will give -10 |
* | Multiplies both operands | A * B will give 200 |
/ | Divides numerator by denominator | B / A will give 2 |
% | Modulus Operator and remainder of after an integer/float division | B % A will give 0 |
The following table shows all the relational operators supported by Swift language. Assume variable A holds 10 and variable B holds 20, then −
Operator | Description | Example |
---|---|---|
== | Checks if the values of two operands are equal or not; if yes, then the condition becomes true. | (A == B) is not true. |
!= | Checks if the values of two operands are equal or not; if values are not equal, then the condition becomes true. | (A != B) is true. |
> | Checks if the value of left operand is greater than the value of right operand; if yes, then the condition becomes true. | (A > B) is not true. |
< | Checks if the value of left operand is less than the value of right operand; if yes, then the condition becomes true. | (A < B) is true. |
>= | Checks if the value of left operand is greater than or equal to the value of right operand; if yes, then the condition becomes true. | (A >= B) is not true. |
<= | Checks if the value of left operand is less than or equal to the value of right operand; if yes, then the condition becomes true. | (A <= B) is true. |
The following table shows all the logical operators supported by Swift language. Assume variable A holds 1 and variable B holds 0 , then −
Operator | Description | Example |
---|---|---|
&& | Called Logical AND operator. If both the operands are non-zero, then the condition becomes true. | (A && B) is false. |
|| | Called Logical OR Operator. If any of the two operands is non-zero, then the condition becomes true. | (A || B) is true. |
! | Called Logical NOT Operator. Use to reverses the logical state of its operand. If a condition is true, then the Logical NOT operator will make it false. | !(A && B) is true. |
Bitwise Operators
Bitwise operators work on bits and perform bit by bit operation. The truth tables for &, |, and ^ are as follows −
p | q | p&q | p|q | p^q |
---|---|---|---|---|
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
1 | 0 | 0 | 1 | 1 |
Assume if A = 60; and B = 13; now in binary format they will be as follows:A = 0011 1100B = 0000 1101-----------------A & B = 0000 1100A|B = 0011 1101A^B = 0011 0001~A = 1100 0011
Bitwise operators supported by Swift language are listed in the following table. Assume variable A holds 60 and variable B holds 13, then −
Operator | Description | Example |
---|---|---|
& | Binary AND Operator copies a bit to the result, if it exists in both operands. | (A & B) will give 12, which is 0000 1100 |
| | Binary OR Operator copies a bit, if it exists in either operand. | (A | B) will give 61, which is 0011 1101 |
^ | Binary XOR Operator copies the bit, if it is set in one operand but not both. | (A ^ B) will give 49, which is 0011 0001 |
~ | Binary Ones Complement Operator is unary and has the effect of 'flipping' bits. | (~A ) will give -61, which is 1100 0011 in 2's complement form. |
<< | Binary Left Shift Operator. The left operands value is moved left by the number of bits specified by the right operand. | (A << 2 will give 240, which is 1111 0000 |
>> | Binary Right Shift Operator. The left operands value is moved right by the number of bits specified by the right operand. | A >> 2 will give 15, which is 0000 1111 |
Assignment Operators
Swift supports the following assignment operators −
Range OperatorsOperator | Description | Example |
---|---|---|
= | Simple assignment operator, Assigns values from right side operands to left side operand | C = A + B will assign value of A + B into C |
+= | Add AND assignment operator, It adds right operand to the left operand and assigns the result to left operand | C += A is equivalent to C = C + A |
-= | Subtract AND assignment operator, It subtracts right operand from the left operand and assigns the result to left operand | C -= A is equivalent to C = C - A |
*= | Multiply AND assignment operator, It multiplies right operand with the left operand and assigns the result to left operand | C *= A is equivalent to C = C * A |
/= | Divide AND assignment operator, It divides left operand with the right operand and assigns the result to left operand | C /= A is equivalent to C = C / A |
%= | Modulus AND assignment operator, It takes modulus using two operands and assigns the result to left operand | C %= A is equivalent to C = C % A |
<<= | Left shift AND assignment operator | C <<= 2 is same as C = C << 2 |
>>= | Right shift AND assignment operator | C >>= 2 is same as C = C >> 2 |
&= | Bitwise AND assignment operator | C &= 2 is same as C = C & 2 |
^= | bitwise exclusive OR and assignment operator | C ^= 2 is same as C = C ^ 2 |
|= | bitwise inclusive OR and assignment operator | C |= 2 is same as C = C | 2 |
Swift includes two range operators, which are shortcuts for expressing a range of values. The following table explains these two operators.
Operator | Description | Example |
---|---|---|
Closed Range | (a...b) defines a range that runs from a to b, and includes the values a and b. | 1...5 gives 1, 2, 3, 4 and 5 |
Half-Open Range | (a..< b) defines a range that runs from a to b, but does not include b. | 1..< 5 gives 1, 2, 3, and 4 |
One- sided Range |
a… , defines a range that runs from a to end of elements
…a , defines a range starting from start to a
|
1… gives 1 , 2,3… end of elements
…2 gives beginning… to 1,2
|
Misc Operators
Swift supports a few other important operators including range and ? : which are explained in the following table.
Operator | Description | Example |
---|---|---|
Unary Minus | The sign of a numeric value can be toggled using a prefixed - | -3 or -4 |
Unary Plus | Returns the value it operates on, without any change. | +6 gives 6 |
Ternary Conditional | Condition ? X : Y | If Condition is true ? Then value X : Otherwise value Y |
Operators Precedence
Operator precedence determines the grouping of terms in an expression. This affects how an expression is evaluated. Certain operators have higher precedence than others; for example, the multiplication operator has higher precedence than the addition operator.
For example, x = 7 + 3 * 2; here, x is assigned 13, not 20 because operator * has higher precedence than +, so it first gets multiplied with 3*2 and then adds into 7.
Here, operators with the highest precedence appear at the top of the table, those with the lowest appear at the bottom. Within an expression, higher precedence operators will be evaluated first.
Operator | Description | Example |
---|---|---|
Primary Expression Operators | () [] . expr++ expr-- | left-to-right |
Unary Operators |
* & + - ! ~ ++expr --expr
* / %
+ -
>> <<
< > <= >=
== !=
| right-to-left |
Binary Operators |
&
^
|
&&
||
| left-to-right |
Ternary Operator | ?: | right-to-left |
Assignment Operators | = += -= *= /= %= >>= <<= &=^= |= | right-to-left |
Comma | , | left-to-right |
Decision Making
language.
What is Decision Making?
This type of program statement requires that the programmer sets several conditions for evaluation within a program. The statement(s) will get executed only if the condition becomes true and optionally, alternative statement or set of statements will get executed if the condition becomes false.
Swift provides the following types of decision-making statements within its programming. They are:-
Sr.No | Statement & Description |
---|---|
1 | if statement
An if statement consists of a Boolean expression followed by one or more statements.
|
2 | if...else statement
An if statement can be followed by an optional else statement, which executes when the Boolean expression is false.
|
3 | if...else if...else Statement
An if statement can be followed by an optional else if...else statement, which is very useful to test various conditions using single if...else if statement.
|
4 | nested if statements
You can use one if or else if statement inside another if or else if statement(s).
|
5 | switch statement
A switch statement allows a variable to be tested for equality against a list of values.
|
An if statement consists of a Boolean expression followed byThe syntax of an if statement in Swift is as follows:
syntax
if boolean_expression { /* statement(s) will execute if the boolean expression is true */ }
If the Boolean expression evaluates to true, then the block of code inside the if statement will be executed. If Boolean
expression evaluates to false, then the first set of code after the end of the if statement (after the closing curly brace) will be executed.
expression evaluates to false, then the first set of code after the end of the if statement (after the closing curly brace) will be executed.
Flow Diagram

Example
var varA:Int = 10 /* Check the boolean condition using if statement */ if varA < 20 { /* If condition is true then print the following */ print("varA is less than 20") } print("Value of variable varA is \(varA)")
OUTPUT
varA is less than 20
Value of variable varA is 10
If-else Statement
OUTPUT
varA is less than 20
Value of variable varA is 10
If-else Statement
An if statement can be followed by an optional else statement,which executes when the Boolean expression is false.
The syntax of an if...else statement in Swift is as follows:if boolean_expression {
/* statement(s) will execute if the boolean expression is true */
} else {
/* statement(s) will execute if the boolean expression is false */
}
If the Boolean expression evaluates to true, then the if block of code will be executed, otherwise else block of code will be executed.
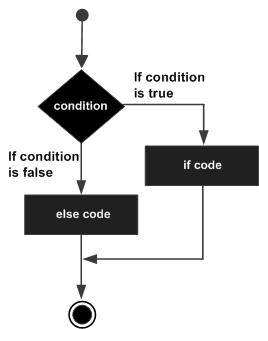
Flow Diagram
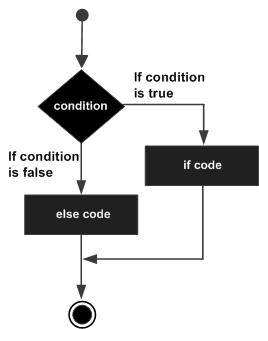
Example
var num:Int = 100 /* Check the boolean condition using if statement */ if num < 20 { /* If condition is true then print the following */ print("Number is less than 20") } else { /* If condition is false then print the following */ print("Number is not less than 20") } print("Value of variable num is \(num)")
OUTPUT
Number is not less than 20
Value of variable num is 100
If…else if…else Statement
An if statement can be followed by an optional else if…else statement, which is very useful to test various conditions
using single if…else if statement.
using single if…else if statement.
When using if, else if, else statements, there are a few points to keep in mind
- An if can have zero or one else’s and it must come after
any else if’s. - An if can have zero to many else if’s and they must come
before the else. - Once an else if succeeds, none of the remaining else if’s
or else’s will be tested
The syntax of an if...else if...else statement in Swift 4 is as follows
if boolean_expression_1 { /* Executes when the boolean expression 1 is true */ } else if boolean_expression_2 { /* Executes when the boolean expression 2 is true */ } else if boolean_expression_3 { /* Executes when the boolean expression 3 is true */ } else { /* Executes when the none of the above condition is true */ }
Example :-
var num:Int = 50 /* Check the boolean condition using if statement */ if num == 20 { /* If condition is true then print the following */ print("Number is equal to than 20") } else if num == 50 { /* If condition is true then print the following */ print("Number is equal to than 50") } else { /* If condition is false then print the following */ print("None of the values is matching") } print("Value of variable num is \(num)")
OUTPUT
Number is equal to than 50 Value of variable num is 50
Nested If Statements
It is always legal in Swift to nest if-else statements, which means you can use one if or else if statement inside another
if or else if statement(s).
if or else if statement(s).
if boolean_expression_1 { /* Executes when the boolean expression 1 is true */ if boolean_expression_2 { /* Executes when the boolean expression 2 is true */ } }
You can nest else if…else in the similar way as you have nested if statement.
import UIKitvar num1:Int = 50
var num2:Int = 60
/* Check the boolean condition using if statement */
if num1 == 50 {
/* If condition is true then print the following */
print("First condition is satisfied")
if num2 == 60 {
/* If condition is true then print the following */
print("Second condition is also satisfied")
}
}
print("Value of variable num1 is \(num1)")
print("Value of variable num2 is \(num2)")
This will output as:-
First condition is satisfied Second condition is also satisfied Value of variable num1 is 50 Value of variable num2 is 60
Switch Statement
A switch statement in Swift 4 completes its execution as soon as the first matching case is completed instead of falling through the bottom of subsequent cases like it happens in C and C++ programming languages. Following is a generic syntax of switch statement in C and C++ −
switch(expression){
case constant-expression :
statement(s);
break; /* optional */
case constant-expression :
statement(s);
break; /* optional */
/* you can have any number of case statements */
default : /* Optional */
statement(s);
}
Following is a generic syntax of switch statement available in Swift
switch expression { case expression1 : statement(s) fallthrough /* optional */ case expression2, expression3 : statement(s) fallthrough /* optional */ default : /* Optional */ statement(s); }
If we do not use fallthrough statement, then the program will come out of switch statement after executing the matching case statement. We will take the following two examples to make its functionality clear.
Example
var index = 10 switch index { case 100 : print("Value of index is 100") case 10,15 : print("Value of index is either 10 or 15") case 5 : print("Value of index is 5") default : print("default case") }
OUTPUT
Value of index is either 10 or 15
Example
var index = 15 switch index { case 100 : print("Value of index is 100") fallthrough case 10,15 : print("Value of index is either 10 or 15") fallthrough case 5 : print("Value of index is 5") default : print("default case") }
OUTPUT
Value of index is either 10 or 15 Value of index is 5
Swift – Loops
In the previous chapters, you have seen that the conditions can be applied to a program to branch the execution of statements within a program. But there may arise some situations where programmers have to repeat the same block of statement(s) several times. For that Swift includes the usual collection of loops and conditional execution features. Swift provides a standard loop constructs such as for, while, do while loop variants.
What are Looping in Swift?
Looping, also known iteration is used in a program to repeat a specific block / segment of code(s). This reduces the tasks of writing the same thing again and again. Here is the flowchart showing how loop statements works:
Flow Diagram
There are various types of loops. These are:
Sr.No | Loop Type & Description |
---|---|
1 | for-in
This loop performs a set of statements for each item in a range, sequence, collection, or progression.
|
2 | while loop
Repeats a statement or group of statements while a given condition is true. It tests the condition before executing the loop body.
|
3 | repeat...while loop
Like a while statement, except that it tests the condition at the end of the loop body.
|
The for-condition-increment loop is functionally the same as the 'for' loop in C. The loop consists of an initialization phase, a test, an increment, and a set of statements that are executed for every iteration of the loop.
Sr.No | Control Statement & Description |
---|---|
1 | continue statement
This statement tells a loop to stop what it is doing and start again at the beginning of the next iteration through the loop.
|
2 | break statement
Terminates the loop statement and transfers execution to the statement immediately following the loop.
|
3 | fallthrough statement
The fallthrough statement simulates the behavior of Swift 4 switch to C-style switch.
|
Syntax:
for initialization; condition; increment {
statements
}
It has three phrases. The initialization phase set up the condition for the start of the loop, i.e. the initialization of loop counter. The second part tests the condition that has been met, whenever this evaluates to true, the statements in the body of the loop are executed once. 3rdly, the increment phase adjusts some variable or value that forms part of the condition test to ensure a stopping condition
for-in loops
You use the for-in loop to iterate over sequences or collections of things, such as the elements of an array or dictionary, the characters in a string, or a range of numbers. Here's the general form is:
Syntax:
for index in sequence {
statements
}
In the above example, this iterates over a range of numbers, the loop index variable (i) takes on the value of the next number in the range each time through the loop:
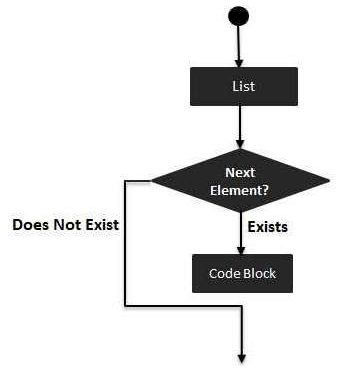
Flow Diagram
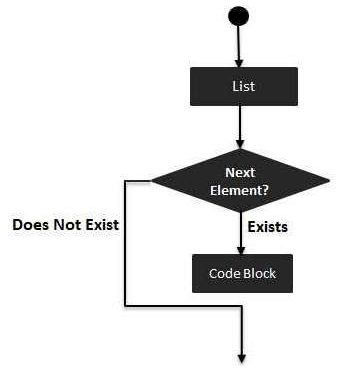
Example
for i in 3...5 {
print ("Value of i is \(i)")
}
OUTPUT
Value of i is 3
Value of i is 4
Value of i is 5
while loops
While loops test the condition ahead of the loop's body and only if the condition evaluates to true then the loop body gets executed. The general format is as follows:
Syntax:
while condition {
statements
}
Here statement(s) may be a single statement or a block of statements. The condition may be any expression. The loop
iterates while the condition is true. When the condition becomes false, the program control passes to the line
immediately following the loop.
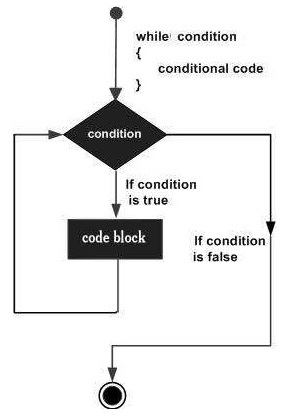
iterates while the condition is true. When the condition becomes false, the program control passes to the line
immediately following the loop.
Flow Diagram
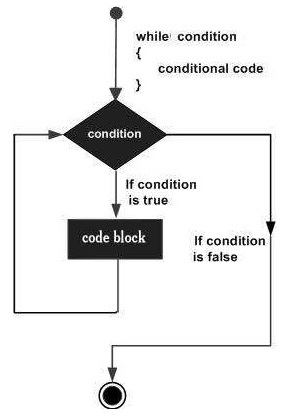
The number 0, the strings ‘0’ and “”, the empty list(), and undef are all false in a Boolean context and all other values
are true. Negation of a true value by ! or not returns a special false value.
are true. Negation of a true value by ! or not returns a special false value.
The key point of a while loop is that the loop might not ever run. When the condition is tested and the result is false, the
loop body will be skipped and the first statement after the while loop will be executed.
loop body will be skipped and the first statement after the while loop will be executed.
import UIKit
var index = 0
while index < 10 {
print("Value of index is \(index)")
index = index + 1
}
Here we are using comparison operator < to compare the value of the variable index against 20. While the value of index is less than 20, the while loop continues executing a block of code next to it and as soon as the value of index becomes equal to 20, it comes out.
When executed, the above code produces the following result:
Value of index is 0 Value of index is 1 Value of index is 2 Value of index is 3 Value of index is 4 Value of index is 5 Value of index is 6 Value of index is 7 Value of index is 8 Value of index is 9
Programmers can use the while loop to replicate the functionality of the for-condition-increment loop, as follows:
Example:
var count = 0
while (count < 8) {
print (count)
count ++
}
In this looping statement, the condition is tested before executing the body of the loop. If the condition evaluates to false for the first time it is executed, the statements in the body of the loop will never execute.
Repeat-While / Do - while Loop
Repeat-while loops test the termination condition at the end of the loop, rather than at the start. This means the statements in the body of the loop are guaranteed to be executed at least once. Loop execution continues until the condition evaluates
to false. The general format for a repeat-while loop looks like this:
Syntax:
repeat {
statements
} while condition
Here's an example of repeat while loop:
Example:
var t = 0
repeat {
print (t)
t++
} while (t < 10)
It is to be noted that the repeat-while loop in Swift 2.0 replaces the do-while
loop from Swift 1.0.
Loop Control Statements
Loop control statements change execution from its normal sequence. When execution leaves a scope, all automatic objects that were created in that scope are destroyed.
Swift supports the following control statements. Click the following links to check their detail.
Sr.No Control Statement & Description
1 continue statement
This statement tells a loop to stop what it is doing and start again at the beginning of the next iteration through the loop.
2 break statement
Terminates the loop statement and transfers execution to the statement immediately following the loop.
3 fallthrough statement
The fallthrough statement simulates the behavior of Swift 4 switch to C-style switch.
Loop control statements change execution from its normal sequence. When execution leaves a scope, all automatic objects that were created in that scope are destroyed.
Sr.No | Control Statement & Description |
---|---|
1 | continue statement
This statement tells a loop to stop what it is doing and start again at the beginning of the next iteration through the loop.
|
2 | break statement
Terminates the loop statement and transfers execution to the statement immediately following the loop.
|
3 | fallthrough statement
The fallthrough statement simulates the behavior of Swift 4 switch to C-style switch.
|
Continue Statement
The continue statement in Swift instructs the loop in which it is for stopping what it is doing and start again at the beginning of the next iteration through the loop.
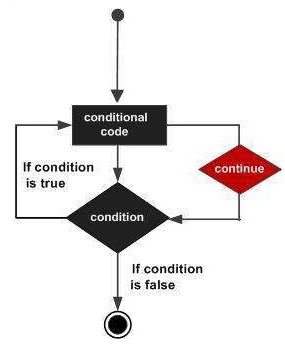
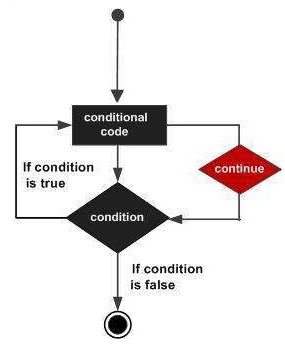
Example:
var index = 10 repeat { index = index + 1 if( index == 12 ){ continue } print("Value of index is \(index)") } while index < 15
OUTPUT
Value of index is 11
Value of index is 13
Value of index is 14
Value of index is 15
Break Statement
The break statement is used to encounter inside a loop. The loop gets terminated at once and the program control resumes at the next statement following the loop.
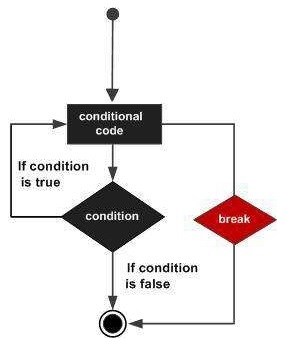
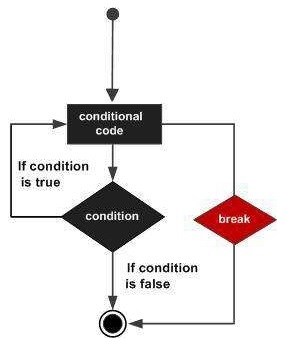
Example:-
var index = 0
repeat {
index = index + 1
if( index == 4 ){
break
}
print( "Value of index is \(index)")
} while index < 10
Value of index is 1 Value of index is 2 Value of index is 3Swift – Fallthrough Statement
A switch statement in Swift completes its execution as soon as the first matching case is completed instead of falling
through the bottom of subsequent cases as it happens in C and C++ programming languages.
through the bottom of subsequent cases as it happens in C and C++ programming languages.
The generic syntax of a switch statement in C and C++ is asfollows:switch(expression){ case constant-expression : statement(s); break; /* optional */ case constant-expression : statement(s); break; /* optional */ /* you can have any number of case statements */ default : /* Optional */ statement(s); }
Here we need to use a break statement to come out of a case statement, otherwise the execution control will fall through
the subsequent case statements available below the matching case statement.
the subsequent case statements available below the matching case statement.
The generic syntax of a switch statement in Swift is asfollows:switch expression { case expression1 : statement(s) fallthrough /* optional */ case expression2, expression3 : statement(s) fallthrough /* optional */ default : /* Optional */ statement(s); }
If we do not use fallthrough statement, then the program will come out of the switch statement after executing the matching case statement. We will take the following two examples to make its functionality clear.
var index = 10 switch index { case 100 : print( "Value of index is 100") case 10,15 : print( "Value of index is either 10 or 15") case 5 : print( "Value of index is 5") default : print( "default case") }This will output as:Value of index is either 10 or 15
var index = 10 switch index { case 100 : print( "Value of index is 100") fallthrough case 10,15 : print( "Value of index is either 10 or 15") fallthrough case 5 : print( "Value of index is 5") default : print( "default case") }This will output as:Value of index is either 10 or 15Value of index is 5
Swift – Strings
Strings in Swift are an ordered collection of characters, such as “Hello, World!” and they are represented by the Swift data type String, which in turn represents a collection of values of Character type.
Create a String
You can create a String either by using a string literal or creating an instance of a String class as follows −
import UIKit // String creation using String literal var str = "Hello, Swift!" print( str ) // String creation using String instance var str2 = String("Hello, Friends!") print( str2 )
When the above code is compiled and executed, it produces the following result −
Hello, Swift!
Hello, Friends!
Empty String
You can create an empty String either by using an empty string literal or creating an instance of String class as shown below. You can also check whether a string is empty or not using the Boolean property isEmpty.
import UIKit // Empty string creation using String literal var str = "" if str.isEmpty { print( "String 1 is empty" ) }else { print( "String 1 is not empty" ) } // Empty string creation using String instance let str2 = String() if str.isEmpty { print( "String 2 is empty" ) }else { print( "String 2 is not empty" ) }
When the above code is compiled and executed, it produces the following result −
String 1 is empty String 2 is empty
String Constants
You can specify whether your String can be modified (or mutated) by assigning it to a variable, or it will be constant by assigning it to a constant using let keyword as shown below −
import UIKit // str can be modified var str = "Hello, Swift!" str + = "--Readers--" print( str ) // str2 can not be modified let str2 = String("Hello, Swift!") str2 + = "--Readers--" print( str2 )
When the above code is compiled and executed, it produces the following result −
Playground execution failed: error: <EXPR>:10:1: error: 'String' is not convertible to '@lvalue UInt8' stringB + = "--Readers--"
String Interpolation
String interpolation is a way to construct a new String value from a mix of constants, variables, literals, and expressions by including their values inside a string literal.
Each item (variable or constant) that you insert into the string literal is wrapped in a pair of parentheses, prefixed by a backslash. Here is a simple example −
import UIKit var varA = 20 let constA = 100 var varC:Float = 20.0 var stringA = "\(varA) times \(constA) is equal to \(varC * 100)" print( stringA )
When the above code is compiled and executed, it produces the following result −
20 times 100 is equal to 2000.0
String Concatenation
You can use the + operator to concatenate two strings or a string and a character, or two characters. Here is a simple example −
import UIKit let constA = "Hello," let constB = "World!" var stringA = constA + constB print(stringA)
When the above code is compiled and executed, it produces the following result −
Hello,World!
String Length
Swift strings do not have a length property, but you can use the global count() function to count the number of characters in a string. Here is a simple example −
import UIKit var varA = "Hello, Swift!" print("\(varA), length is \(count(varA))")
When the above code is compiled and executed, it produces the following result −
Hello, Swift!, length is 13
String Comparison
You can use the == operator to compare two strings variables or constants. Here is a simple example −
import UIKit var varA = "Hello, Swift!" var varB = "Hello, World!" if varA == varB { print( "\(varA) and \(varB) are equal" ) }else { print( "\(varA) and \(varB) are not equal" ) }
When the above code is compiled and executed, it produces the following result −
Hello, Swift! and Hello, World! are not equal
Unicode Strings
You can access a UTF-8 and UTF-16 representation of a String by iterating over its utf8 and utf16 properties as demonstrated in the following example −
import UIKit var unicodeString = "Dog‼🐶" print("UTF-8 Codes:") for code in unicodeString.utf8 { print("\(code) ") } print("\n") print("UTF-16 Codes: ") for code in unicodeString.utf16 { print("\(code) ") }
When the above code is compiled and executed, it produces the following result −
UTF-8 Codes:
68 111 103 226 128 188 240 159 144 182
UTF-16 Codes:
68 111 103 8252 55357 56374
String Functions & Operators
Swift supports a wide range of methods and operators related to Strings −
Sr.No | Functions/Operators & Purpose |
---|---|
1 |
isEmpty
A Boolean value that determines whether a string is empty or not.
|
2 |
hasPrefix(prefix: String)
Function to check whether a given parameter string exists as a prefix of the string or not.
|
3 |
hasSuffix(suffix: String)
Function to check whether a given parameter string exists as a suffix of the string or not.
|
4 |
toInt()
Function to convert numeric String value into Integer.
|
5 |
count()
Global function to count the number of Characters in a string.
|
6 |
utf8
Property to return a UTF-8 representation of a string.
|
7 |
utf16
Property to return a UTF-16 representation of a string.
|
8 |
unicodeScalars
Property to return a Unicode Scalar representation of a string.
|
9 |
+
Operator to concatenate two strings, or a string and a character, or two characters.
|
10 |
+=
Operator to append a string or character to an existing string.
|
11 |
==
Operator to determine the equality of two strings.
|
12 |
<
Operator to perform a lexicographical comparison to determine whether one string evaluates as less than another.
|
13 |
startIndex
To get the value at starting index of string.
|
14 |
endIndex
To get the value at ending index of string.
|
15 |
Indices
To access the indeces one by one. i.e all the characters of string one by one.
|
16 |
insert("Value", at: position)
To insert a value at a position.
|
17 |
remove(at: position)
removeSubrange(range)
to remove a value at a position, or to remove a range of values from string.
|
18 |
reversed()
returns the reverse of a string
|
Swift – Characters
A character in Swift is a single character String literal, addressed by the data type character. Take a look at the following example. It uses two Character constants −
import UIKit
let char1: Character = "A"
let char2: Character = "B"
print("Value of char1 \(char1)")
print("Value of char2 \(char2)")
When the above code is compiled and executed, it produces the following result −
Value of char1 A
Value of char2 B
If you try to store more than one character in a Character type variable or constant, then Swift will not allow that. Try to type the following example in Swift Playground and you will get an error even before compilation.
import UIKit
// Following is wrong in Swift
let char: Character = "AB"
print("Value of char \(char)")
Empty Character Variables
It is not possible to create an empty Character variable or constant which will have an empty value. The following syntax is not possible −
import UIKit
// Following is wrong in Swift
let char1: Character = ""
var char2: Character = ""
print("Value of char1 \(char1)")
print("Value of char2 \(char2)")
Accessing Characters from Strings
As explained while discussing Swift’s Strings, String represents a collection of Character values in a specified order. So we can access individual characters from the given String by iterating over that string with a for-in loop −
import UIKit for ch in "Hello" { print(ch) }
When the above code is compiled and executed, it produces the following result −
H
e
l
l
o
Concatenating Strings with Characters
The following example demonstrates how a Swift’s Character can be concatenated with Swift’s String.
var str:String = "Hello "
let char:Character = "G"
str.append( char )
print("Value of new String = \(str)")
When the above code is compiled and executed, it produces the following result −
Value of new String = Hello G
Swift – Arrays
Swift 4 arrays are used to store ordered lists of values of the same type. Swift 4 puts strict checking which does not allow you to enter a wrong type in an array, even by mistake.
If you assign a created array to a variable, then it is always mutable, which means you can change it by adding, removing, or changing its items; but if you assign an array to a constant, then that array is immutable, and its size and contents cannot be changed.
Creating Arrays
You can create an empty array of a certain type using the following initializer syntax −
var someArray = [SomeType]()
Here is the syntax to create an array of a given size a* and initialize it with a value −
var someArray = [SomeType](count: NumbeOfElements, repeatedValue: InitialValue)
You can use the following statement to create an empty array of Int type having 3 elements and the initial value as zero −
var someInts = [Int](count: 3, repeatedValue: 0)
Following is one more example to create an array of three elements and assign three values to that array −
var someInts:[Int] = [10, 20, 30]
Accessing Arrays
You can retrieve a value from an array by using subscript syntax, passing the index of the value you want to retrieve within square brackets immediately after the name of the array as follows −
var someVar = someArray[index]
Here, the index starts from 0 which means the first element can be accessed using the index as 0, the second element can be accessed using the index as 1 and so on. The following example shows how to create, initialize, and access arrays −
var someInts = [Int](count: 3, repeatedValue: 10) var someVar = someInts[0] print( "Value of first element is \(someVar)" ) print( "Value of second element is \(someInts[1])" ) print( "Value of third element is \(someInts[2])" )
When the above code is compiled and executed, it produces the following result −
Value of first element is 10
Value of second element is 10
Value of third element is 10
Modifying Arrays
You can use append() method or addition assignment operator (+=) to add a new item at the end of an array. Take a look at the following example. Here, initially, we create an empty array and then add new elements into the same array −
var someInts = [Int]() someInts.append(20) someInts.append(30) someInts += [40] var someVar = someInts[0]print( "Value of first element is \(someVar)" ) print( "Value of second element is \(someInts[1])" ) print( "Value of third element is \(someInts[2])" )
When the above code is compiled and executed, it produces the following result −
Value of first element is 20
Value of second element is 30
Value of third element is 40
You can modify an existing element of an Array by assigning a new value at a given index as shown in the following example −
var someInts = [Int]() someInts.append(20) someInts.append(30) someInts += [40] // Modify last element someInts[2] = 50 va someVar = someInts[0] print( "Value of first element is \(someVar)" ) print( "Value of second element is \(someInts[1])" ) print( "Value of third element is \(someInts[2])" )
When the above code is compiled and executed, it produces the following result −
Value of first element is 20
Value of second element is 30
Value of third element is 50
Iterating Over an Array
You can use for-in loop to iterate over the entire set of values in an array as shown in the following example −
var someStrs = [String]() someStrs.append("C") someStrs.append("C++") someStrs += ["Swift"] for item in someStrs { print(item) }
When the above code is compiled and executed, it produces the following result −
C
C++
Swift
You can use enumerate() function which returns the index of an item along with its value as shown below in the following example −
var someStrs = [String]() someStrs.append("C") someStrs.append("C++") someStrs += ["Swift"] for (index, item) in someStrs.enumerated() { print("Value at index = \(index) is \(item)") }
When the above code is compiled and executed, it produces the following result −
Value at index = 0 is C
Value at index = 1 is C++
Value at index = 2 is Swift
Adding Two Arrays
You can use the addition operator (+) to add two arrays of the same type which will yield a new array with a combination of values from the two arrays as follows −
var intsA = [Int](count:2, repeatedValue: 2) var intsB = [Int](count:3, repeatedValue: 1) var intsC = intsA + intsB for item in intsC { print(item) }
When the above code is compiled and executed, it produces the following result −
2
2
1
1
1
The count Property
You can use the read-only count property of an array to find out the number of items in an array shown below −
var intsA = [Int](count:2, repeatedValue: 2) var intsB = [Int](count:3, repeatedValue: 1) var intsC = intsA + intsB print("Total items in intsA = \(intsA.count)") print("Total items in intsB = \(intsB.count)") print("Total items in intsC = \(intsC.count)")
When the above code is compiled and executed, it produces the following result −
Total items in intsA = 2
Total items in intsB = 3
Total items in intsC = 5
The empty Property
You can use the read-only empty property of an array to find out whether an array is empty or not as shown below −
var intsA = [Int](count:2, repeatedValue: 2) var intsB = [Int]() var intsC = [Int]() print("intsA.isEmpty = \(intsA.isEmpty)") print("intsB.isEmpty = \(intsB.isEmpty)") print("intsC.isEmpty = \(intsC.isEmpty)")
When the above code is compiled and executed, it produces the following result −
intsA.isEmpty = false intsB.isEmpty = true intsC.isEmpty = true
Its V good . 😘😘❤️ exam ma help krse
ReplyDelete